Optimizing Selenium Test Scripts for Safari on Windows Platform

Since Selenium is an open-source framework with a strong community and extensive plugins, it offers robust support for various browsers. However, Safari for Windows, discontinued by Apple Inc. in 2012, poses a challenge. Writing Selenium test scripts for Safari on Windows requires special considerations due to its outdated nature and potential compatibility issues, which can impact the consistency of user experience across different environments.
This article will explore Safari for Windows and how to optimize the Selenium test scripts for Safari.
What Is Selenium?
Selenium is an automation testing tool that checks if they perform as expected. It is a popular choice among testers for browser testing. Selenium is platform-independent, allowing for distributed testing using the Selenium Grid. It is a powerful tool for controlling web browsers through programming and performing browser automation. Selenium supports all major browsers, works on all major operating systems, and has scripts that can be written in various programming languages.
Setting Selenium for Safari on Windows
Though setting up Safari for Windows is possible, it’s important to note that it is no longer supported or updated by Apple for any Windows system, which could pose security risks.
Installing Safari on Windows
1.Download Safari 5.1.7 Installer:
You must find a third-party source or website since the official download is no longer available from Apple through its website or product stores.
Search for “Safari 5.1.7 for Windows download” on your preferred search engine. Safari 5.1.7 was the last version released for Windows in 2010 and is outdated.
- Choose a safe site to download the installer. Some websites often host older software versions, but always be cautious and make sure you go through all the privacy policies before ticking them up.
2.Install Safari:
- Run the Installer:
- Once you have downloaded the installer file, locate it on your system (usually in the “Downloads” folder).Now, double-click the installer to begin the installation process.
Installing Selenium WebDriver
To interact with Safari, Selenium WebDrivers should be installed on Windows.
Setting up Selenium involves two components: the Selenium package for Python or any language supported by Selenium and the driver for the browser you want to use.
Selenium Package:
Firstly, to download the Selenium package, execute the pip command in your terminal:
Configuring SafariDriver
SafariDriver controls Safari browsers. You might encounter compatibility issues since SafariDriver for Windows is not officially supported. A possible workaround is using the Remote WebDriver with Selenium Grid.
- Set up a Selenium Grid hub and node.
- Configure the node to use SafariDriver.
- Connect to the grid from your test script using Remote WebDriver.
Example Python script:
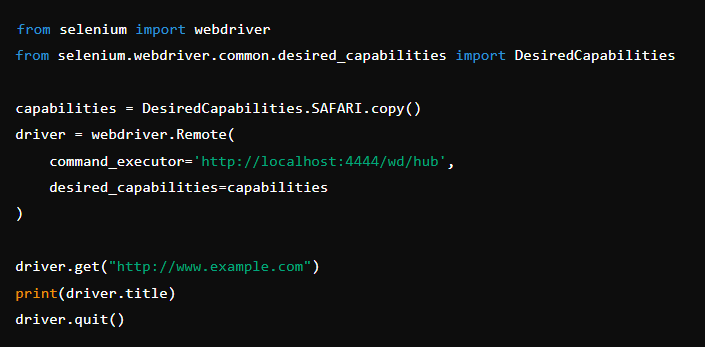
Optimization Techniques for Selenium Test Scripts on Safari
Following are the optimization techniques for Selenium test scripts:
Handling Browser Timeouts and Synchronization
One of the common challenges when running Selenium tests on Safari is managing timeouts and synchronization issues. Safari’s rendering engine and event handling mechanisms may differ from other browsers, leading to potential timing discrepancies during test execution. To mitigate this, consider the following strategies:
- Instead of relying on fixed delays or sleep statements, utilize explicit wait conditions in your Selenium scripts. This approach waits for specific conditions (e.g., element visibility, presence, or state change) before proceeding with subsequent actions, ensuring accurate timing and reducing the risk of test failures due to synchronization issues.
- Experiment with increasing or decreasing timeout values for specific operations, such as page loads, element interactions, or script execution. Finding the optimal timeout settings can improve test stability and reduce false positives caused by premature timeouts.
Handling Safari-specific Exceptions and Errors
Safari may exhibit unique behavior or generate specific exceptions and errors during test execution. To handle these scenarios effectively, consider the following strategies:
- Implement robust exception handling: Enhance your Selenium scripts with comprehensive exception-handling mechanisms that can gracefully handle and recover from Safari-specific exceptions. This may involve catching and handling specific exception types, logging relevant information for debugging purposes, or implementing retry mechanisms for flaky tests.
Optimizing Performance
Safari’s rendering engine and resource utilization patterns may differ from other browsers, potentially impacting the performance of your Selenium tests. To optimize performance, consider the following techniques:
- Implement parallel test execution: Leverage Selenium’s capability to run tests in parallel, effectively distributing the workload across multiple threads or processes. This can significantly reduce test execution time, especially for large test suites or resource-intensive scenarios.
- Utilize cloud-based testing platforms: Consider leveraging cloud-based testing platforms that offer dedicated Safari instances on Windows machines. These platforms often provide optimized environments and infrastructure, ensuring reliable and consistent test execution.
Handling Browser-specific Quirks and Limitations
Each browser may exhibit unique quirks or limitations that require special handling within your Selenium scripts. For Safari on Windows, consider the following strategies:
- Implement browser-specific workarounds: Maintain a catalog of known browser-specific quirks or limitations and implement appropriate workarounds within your Selenium scripts. This may involve writing custom helper functions, applying alternative locator strategies, or implementing browser-specific logic to handle these scenarios gracefully.
- Leverage browser-specific capabilities: Investigate and leverage browser-specific capabilities provided by Selenium for Safari. These capabilities can offer additional control or functionality tailored to the Safari browser, potentially simplifying or enhancing certain test scenarios.
Leveraging Community Resources
The Selenium community is a valuable source of knowledge and best practices. Consider the following strategies to leverage community resources:
- Participate in forums and online communities: Engage with the Selenium community through forums, mailing lists, or online discussion groups. Share your experiences, seek guidance, and contribute to the collective knowledge base, particularly regarding Safari-specific challenges and solutions.
- Stay updated with Selenium releases and documentation: Regularly review the release notes and documentation provided by the Selenium project. New versions may introduce improvements, bug fixes, or enhanced features that can positively impact your Safari testing efforts on Windows.
Best Practices for Writing Selenium Test Scripts
One of the main reasons Selenium testing is so widely used across different platforms is the wide range of options it provides with tools and different libraries. With every increment in tool options, choosing the right tool for the right job is crucial. To overcome this hurdle, we can follow some basic practices and patterns that make the whole selenium testing process more efficient and organized.
Page object models: The Page object design is like representing a web page using an extraordinary dialect. Dialect makes a difference in stowing away how the page works. Instead of specifically connecting with a page, tests utilize classes and strategies to do the same thing. This design stows away points of interest, like IDs, and makes a system, particularly for the tested application.
Stabilizing the Tests: To maintain the reliability and effectiveness of selenium testing, some crucial key points should be considered while working on the tests or test codes. A few of these key points are:
- Maintaining a stable test suite.
- Discarding bad code changes.
- Regular Maintenance.
- Use of explicit waits.
- Reporting of failures.
Fresh browser per test: Every test case should have a clean environment to run the stimulation as it ensures the isolation and reliability of the testing without any cross-interference. Some of the browsers start each test with a clean environment by default.
Test Independence: Writing every complex test code is a work of art. Still, along with it, it is very important to make sure these different test codes don’t interfere with the running of other tests and give accurate results without relying on other test outcomes or their previous state data. This will not only slow down the whole process but will also increase the complexity of the code, making its readability bad as well. It is always better to create separate test cases, which also gives organized and efficient work. When test cases are designed in such a way that they are independent, they are easier to debug and maintain.
Domain-specific language: Domain-specific language(DSL) in Selenium testing is a customized language designed to fill the gap between the testers and the web application users. DSL suggests that rather than using technical terms, the web application should contain more friendly terms, making the user experience better and more stable.
It also makes it easier for non-technical users to understand and contribute to test automation efficiently.
DSL can be implemented in selenium testing in two simple steps:
- Defining the Domain-specific language commands: This is done by creating a class and encapsulating the commands.
- Using the Domain-specific language commands in the test scripts.
Use of the right locators: In selenium testing, locators serve as a pointer to web elements of any web application, like links, checkboxes, and text fields, and they also control the automation like clicking a certain button, typing, or reading some data. Selenium provides and supports multiple kinds of locators, some of which are:
- ID Locator
- Name Locator
- Class Name Locator
- Tag Name Locator
- Link Text Locator
- CSS Selector Locator
- Partial Link Text Locator
- XPath Locator
How To Run Safari on Windows?
Due to Safari’s lack of official support on the Windows platform, optimizing the Selenium test script is a challenge. However, using the right advanced techniques and workarounds can enhance the testing strategy.
If you specifically need to test websites for Safari browser online compatibility on a Windows machine, consider these options:
- Virtual Machines: Set up a macOS virtual machine on your Windows PC to run Safari.
- Cloud Testing tools: Use online services like LambdaTest to test websites on various browsers, including Safari. LambdaTest is an AI-powered test orchestration and execution platform that lets you run manual and automated tests at scale with over 3000+ real devices, browsers, and OS combinations.
- Responsive Design Mode: Most modern browsers have tools to simulate different screen sizes and devices, which can partially substitute for browser-specific testing.
Conclusion
Optimizing any test script using Selenium or any other tool always requires creativity and advanced techniques. Doing the same on Safari browsers becomes more complex due to its inherent limitations and lack of direct support. Still, testing on Safari remains crucial due to its user base. Despite all the setbacks and challenges, some techniques and tools make testing on Safari possible and efficient.
The future of browser testing is evolving with additional resources and technologies. Through planning, strategic use of technology, and a commitment to best practices, the testing experience on Safari for Windows will only get better.